How to Use the Geometry Dash Detection API
Geometry Dash is an exciting game filled with rhythm-based challenges. But did you know you can use the Geometry Dash Detection API to enhance the experience? Whether you’re a developer or just curious, this guide will help you understand and use it easily.
What is the Geometry Dash Detection API?
Table of Contents
The Geometry Dash Detection API is a tool that lets you collect and use data from the game. It can help detect player actions, levels, scores, and more. This is useful for developers who want to create bots, mods, or tracking tools.
Why Use This API?
- Track player progress – Get real-time data from the game.
- Create custom tools – Build mods or bots to enhance gameplay.
- Analyze game stats – Find patterns and improve your skills.
Getting Started
Before using the API, you need some basic coding knowledge. Don’t worry, though – you don’t have to be an expert!
Step 1: Get API Access
First, check if the API is public or if you need an API key. Some versions of the API may require authentication.
Step 2: Make Your First Request
To start using the API, you need to send a GET request. Here’s an example in Python:
import requests url = "https://geometrydashapi.com/detect" response = requests.get(url) print(response.json())
This code retrieves data from the API and prints it in a readable format.
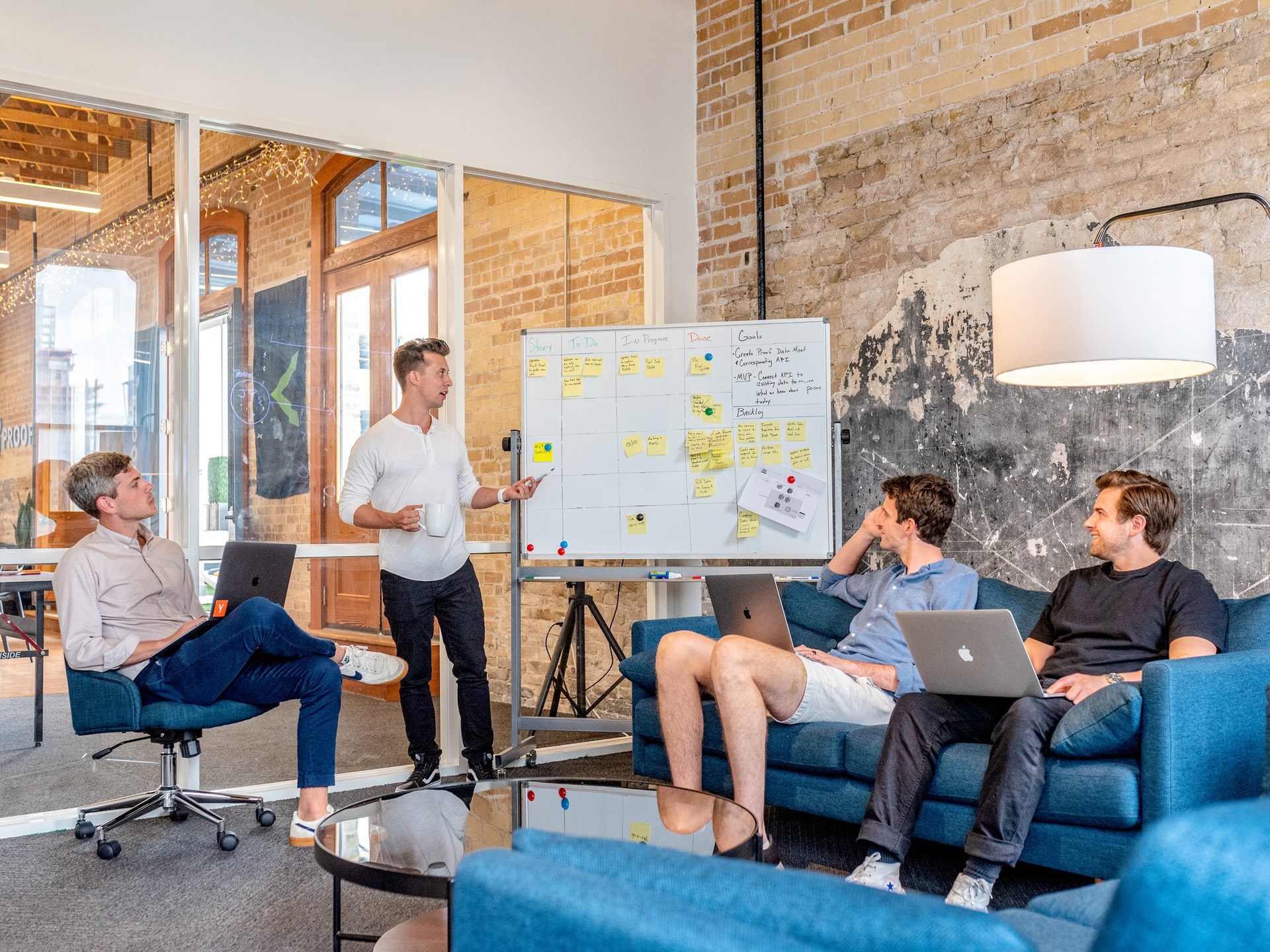
Understanding the Response
When you call the API, it sends back a response in JSON format. Here’s an example:
{ "player": "CoolGamer99", "level": "Electrodynamix", "attempts": 25, "status": "Playing" }
In this response, you see the player’s name, the level they are on, how many times they’ve tried, and their current status.
Step 3: Use the Data
Now that you have data, you can do lots of cool things with it!
- Display player stats on a website.
- Create alerts when a player reaches a milestone.
- Analyze game trends over time.
Advanced Usage
Once you’re comfortable with basic requests, try using more API features:
Filtering Data
You can request specific details by adding parameters. For example, you might want to check only a specific level:
url = "https://geometrydashapi.com/detect?level=Electrodynamix"
Automating Requests
If you want real-time updates, set up a loop:
import time while True: response = requests.get(url) print(response.json()) time.sleep(5) # Wait 5 seconds before checking again
This script checks for updates every five seconds.
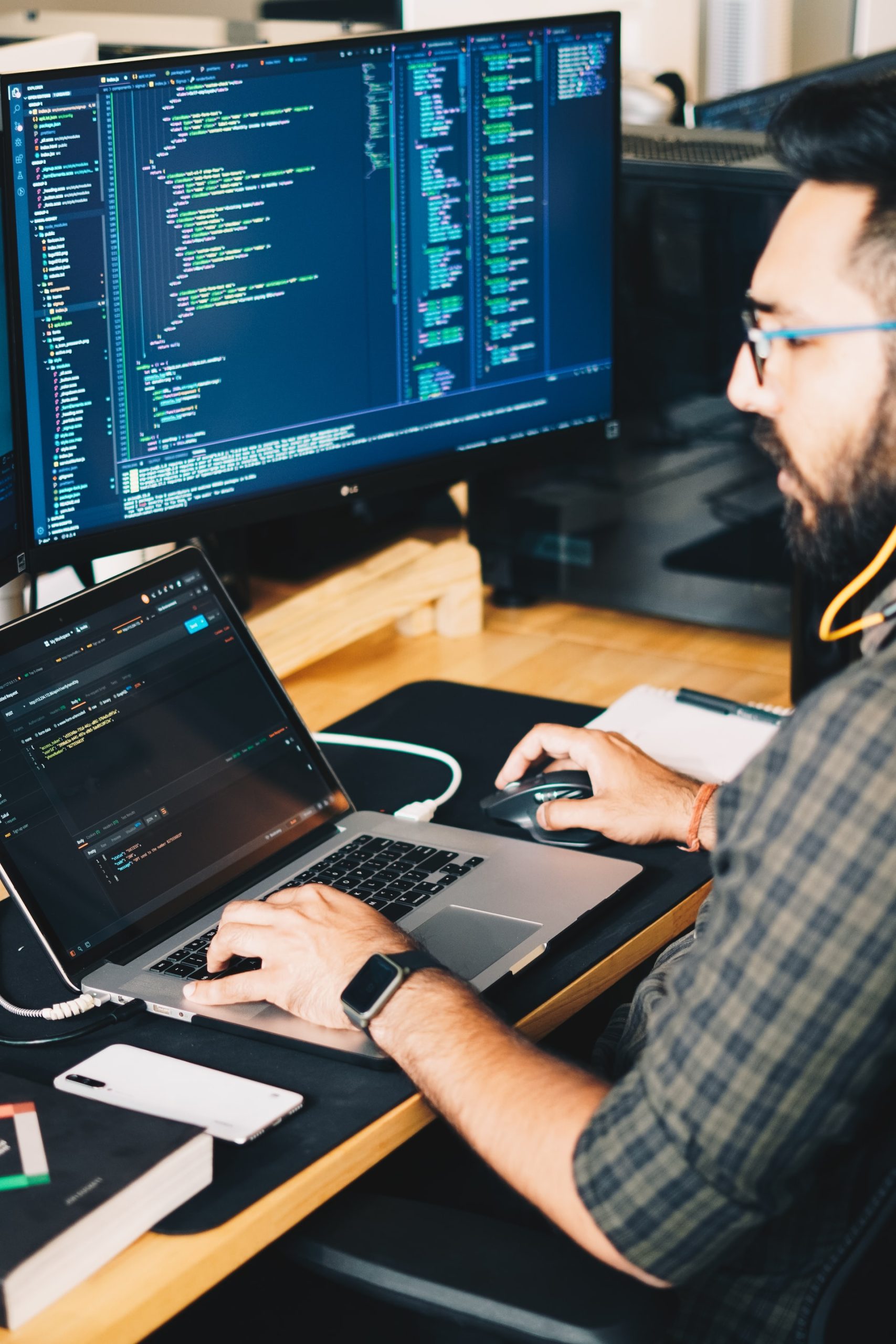
Common Issues and Fixes
Getting No Response
If the API doesn’t respond, check:
- If the URL is correct.
- If you have an API key (if required).
- If the server is down.
Data Not Updating
Sometimes, the API might cache old data. Try:
- Waiting a few seconds and retrying.
- Checking if a new request is necessary.
- Refreshing your connection.
Final Thoughts
The Geometry Dash Detection API is a powerful tool. With some creativity, you can develop awesome projects, track progress, or even make new game features.
Have fun experimenting! The more you practice, the better your results will be.